parent
8912f1f03d
commit
9f94dfb45f
56
README.md
56
README.md
|
@ -1,19 +1,6 @@
|
||||||
# Y—Catalog-Viewer
|
# Y—Catalog-Viewer
|
||||||
A standalone catalog viewer package that intelligently displays design components.
|
A standalone catalog viewer package that intelligently displays design components.
|
||||||
|
|
||||||
Requirements
|
|
||||||
----------
|
|
||||||
|
|
||||||
### Jazzy (documentation)
|
|
||||||
```
|
|
||||||
sudo gem install jazzy
|
|
||||||
```
|
|
||||||
|
|
||||||
Setup
|
|
||||||
----------
|
|
||||||
|
|
||||||
Open `Package.swift` in Xcode.
|
|
||||||
|
|
||||||
|
|
||||||
### Sample code to display built-in components
|
### Sample code to display built-in components
|
||||||
|
|
||||||
|
@ -43,10 +30,12 @@ where the user needs to provide the following parameters:
|
||||||
* The detail label which is an optional parameter, allows a user to include additional details about the image if needed.
|
* The detail label which is an optional parameter, allows a user to include additional details about the image if needed.
|
||||||
* The axis attribute which is an optional parameter with which a user can decide whether they want to display the title, details, and image to be displayed vertically or horizontally.
|
* The axis attribute which is an optional parameter with which a user can decide whether they want to display the title, details, and image to be displayed vertically or horizontally.
|
||||||
|
|
||||||
|
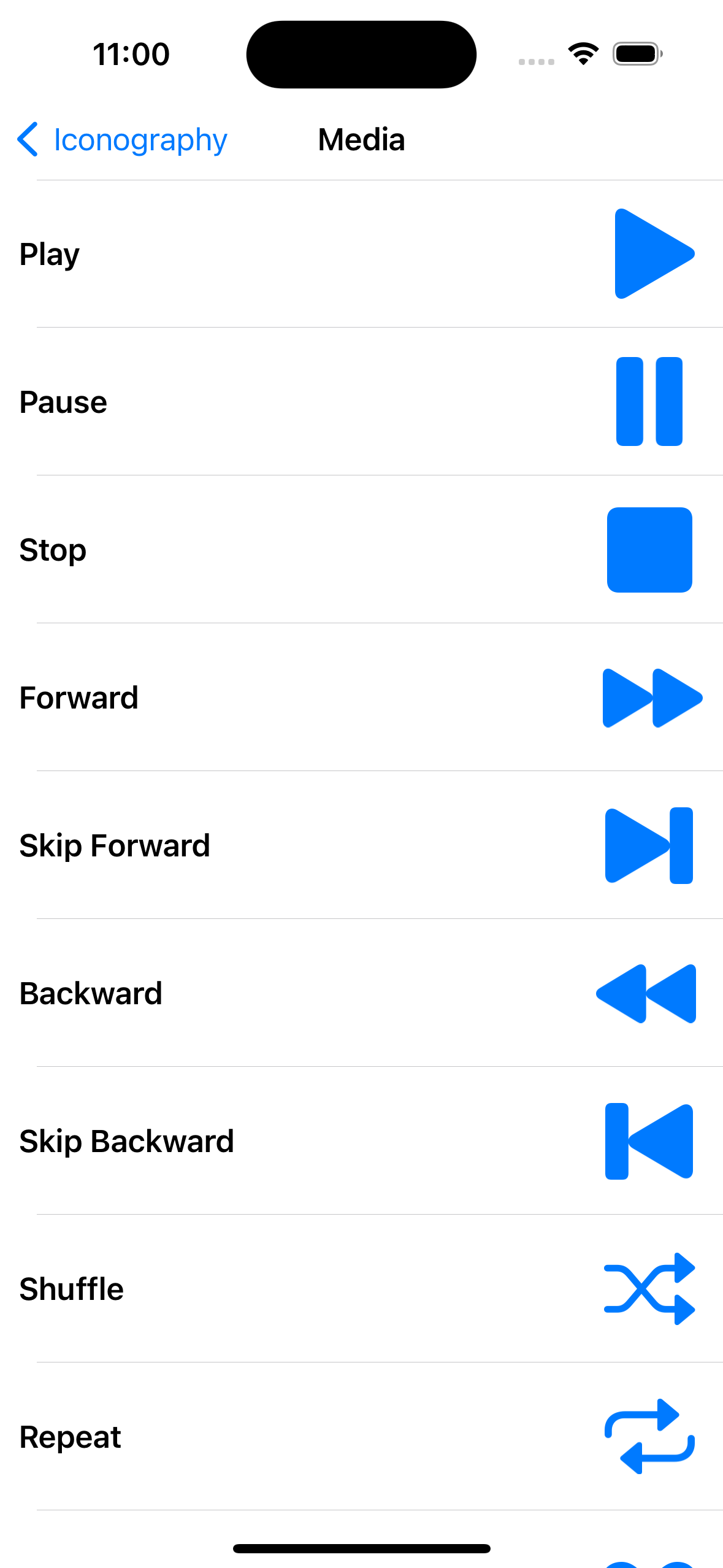
|
||||||
|
|
||||||
|
|
||||||
a user can create a font category as follows:
|
a user can create a font category as follows:
|
||||||
```
|
```
|
||||||
enum TypographySample {
|
enum FontSample {
|
||||||
static var tiemposHeadlineBold: FontCategory {
|
static var tiemposHeadlineBold: FontCategory {
|
||||||
FontCategory(
|
FontCategory(
|
||||||
name: "TiemposHeadline Bold",
|
name: "TiemposHeadline Bold",
|
||||||
|
@ -61,7 +50,7 @@ where the user needs to provide the following parameters:
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
where the user needs to provide the following parameters:
|
where the user needs to provide the following parameters:
|
||||||
* The name of the category.
|
* The name of the category.
|
||||||
* Model for the font to be displayed, which again includes:
|
* Model for the font to be displayed, which again includes:
|
||||||
|
@ -69,10 +58,13 @@ where the user needs to provide the following parameters:
|
||||||
* model which is the font for the title and detail label.
|
* model which is the font for the title and detail label.
|
||||||
* The detail label which is an optional parameter, allows a user to display additional details if needed.
|
* The detail label which is an optional parameter, allows a user to display additional details if needed.
|
||||||
* The axis attribute which is an optional parameter with which a user can decide whether they want to display the title and details to be displayed vertically or horizontally.
|
* The axis attribute which is an optional parameter with which a user can decide whether they want to display the title and details to be displayed vertically or horizontally.
|
||||||
|
|
||||||
|
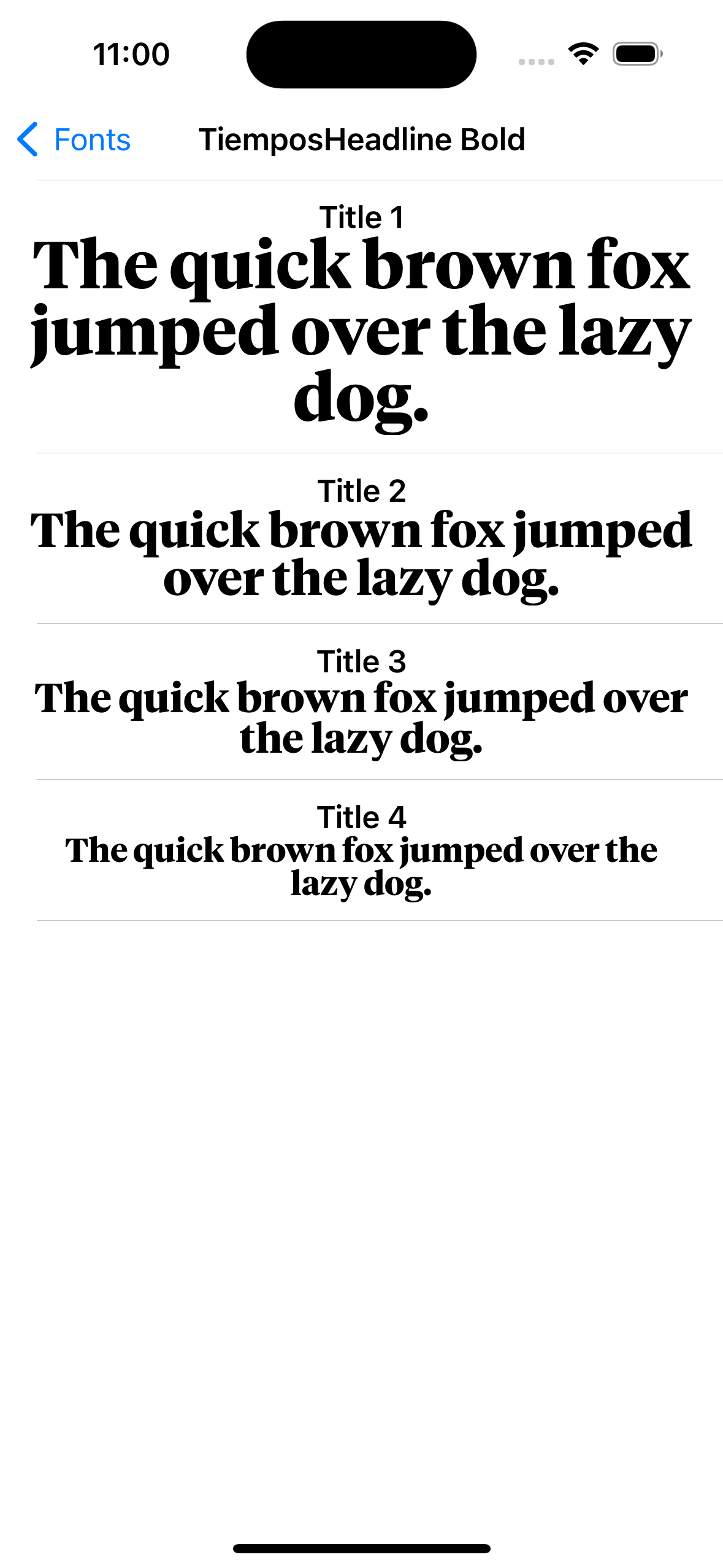
|
||||||
|
|
||||||
|
|
||||||
a user can create a color category as follows:
|
a user can create a color category as follows:
|
||||||
```
|
```
|
||||||
enum colourSample {
|
enum ColorSample {
|
||||||
static var colorSample: ColorCategory {
|
static var colorSample: ColorCategory {
|
||||||
ColorCategory(
|
ColorCategory(
|
||||||
name: "Easter",
|
name: "Easter",
|
||||||
|
@ -95,6 +87,9 @@ where the user needs to provide the following parameters:
|
||||||
* The detail label which is an optional parameter, allows a user to display additional details if needed.
|
* The detail label which is an optional parameter, allows a user to display additional details if needed.
|
||||||
* The axis attribute which is an optional parameter with which a user can decide whether they want to display the title, details and color view to be displayed vertically or horizontally.
|
* The axis attribute which is an optional parameter with which a user can decide whether they want to display the title, details and color view to be displayed vertically or horizontally.
|
||||||
|
|
||||||
|
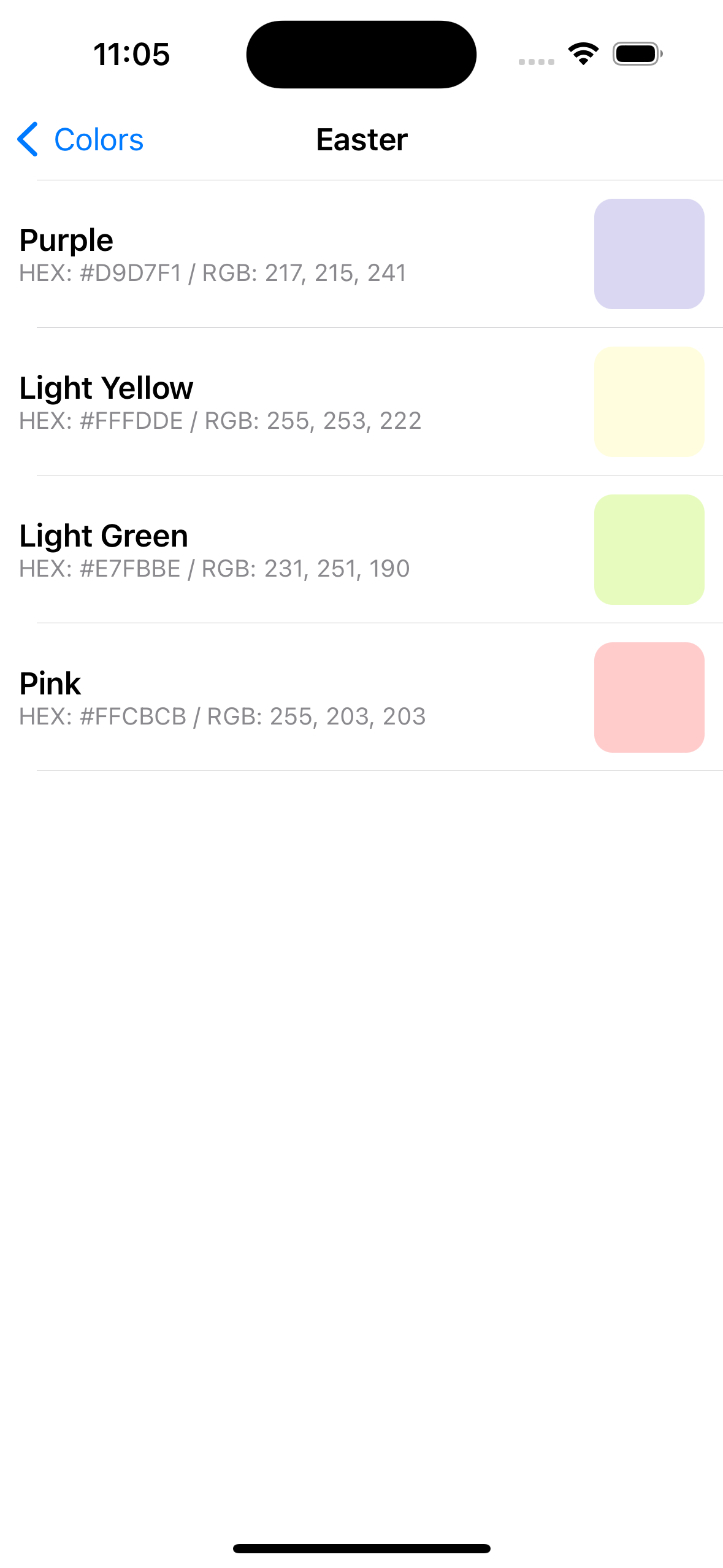
|
||||||
|
|
||||||
|
|
||||||
We can create a category which contains other categories.
|
We can create a category which contains other categories.
|
||||||
```
|
```
|
||||||
let foundationalCategory = CatalogCategory(
|
let foundationalCategory = CatalogCategory(
|
||||||
|
@ -102,7 +97,7 @@ We can create a category which contains other categories.
|
||||||
subcategories: [
|
subcategories: [
|
||||||
ColorSample.category,
|
ColorSample.category,
|
||||||
IconographySample.category,
|
IconographySample.category,
|
||||||
TypographySample.category
|
FontSample.category
|
||||||
]
|
]
|
||||||
)
|
)
|
||||||
```
|
```
|
||||||
|
@ -110,6 +105,8 @@ We can create a category which contains other categories.
|
||||||
* The name of the category.
|
* The name of the category.
|
||||||
* Array of subcategories.
|
* Array of subcategories.
|
||||||
|
|
||||||
|
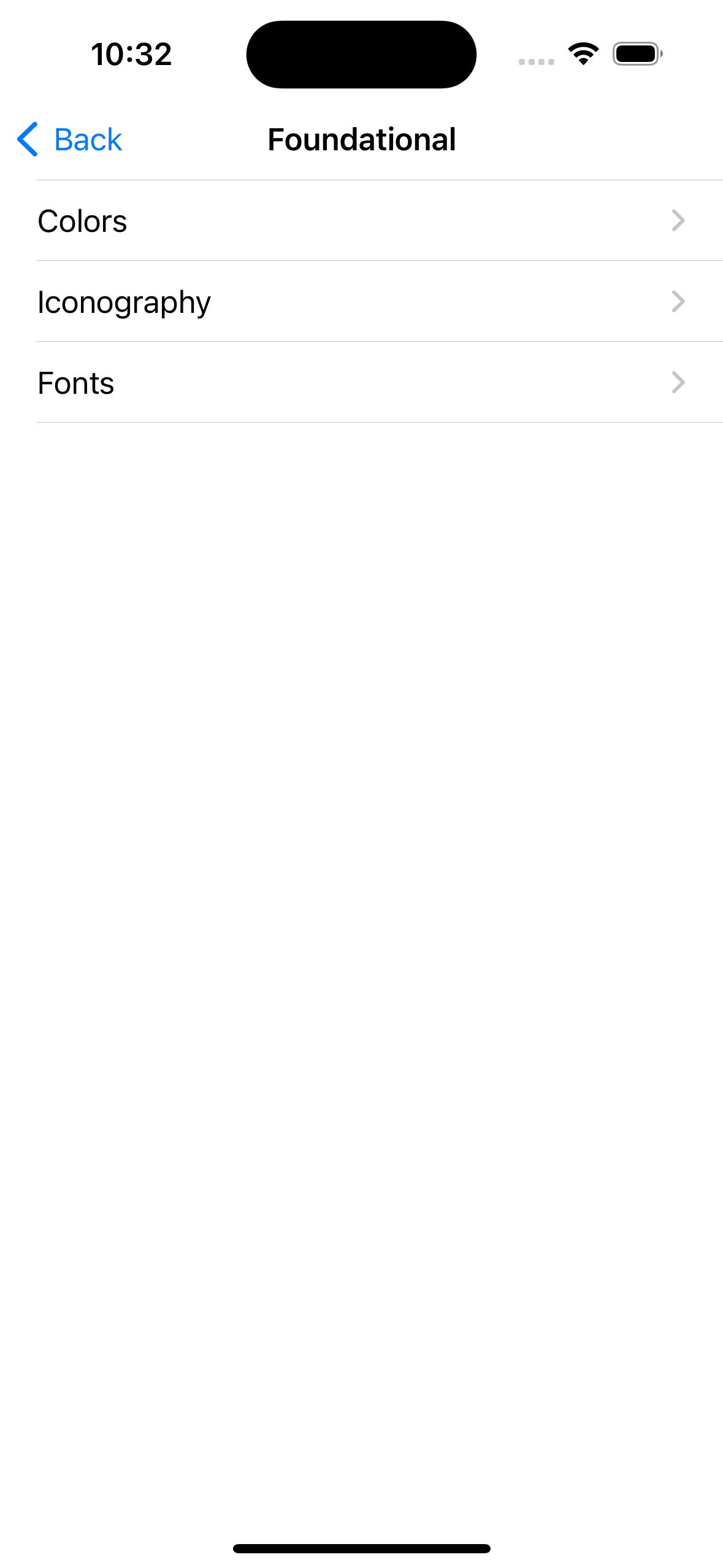
|
||||||
|
|
||||||
|
|
||||||
### Sample code to display Custom Components
|
### Sample code to display Custom Components
|
||||||
|
|
||||||
|
@ -140,6 +137,8 @@ enum DemoButtonSample {
|
||||||
* name for the category.
|
* name for the category.
|
||||||
* model needed to initialise the button.
|
* model needed to initialise the button.
|
||||||
|
|
||||||
|
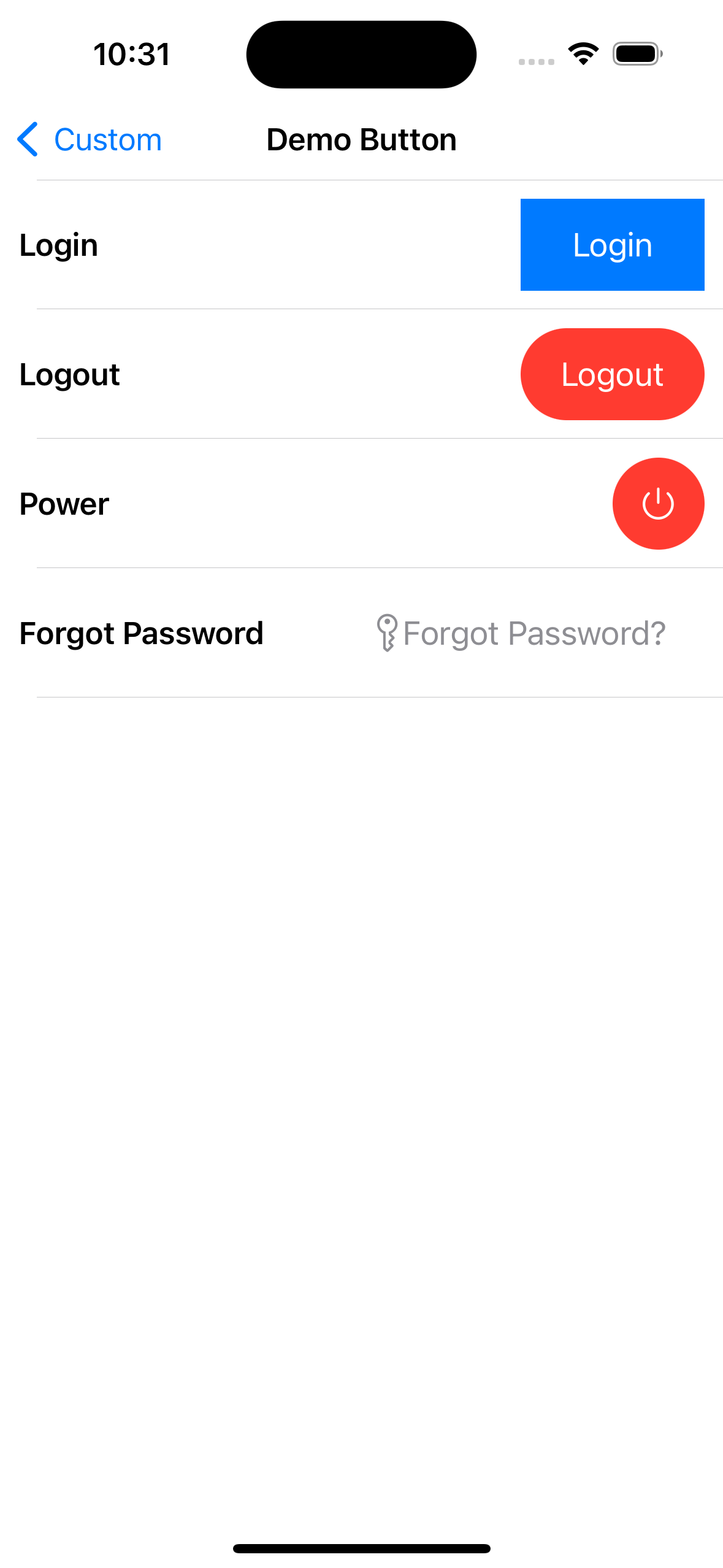
|
||||||
|
|
||||||
|
|
||||||
We can display a custom view within the catalog.
|
We can display a custom view within the catalog.
|
||||||
Suppose a user wants to display a custom view named DemoView from their project.
|
Suppose a user wants to display a custom view named DemoView from their project.
|
||||||
|
@ -163,6 +162,9 @@ enum DemoButtonSample {
|
||||||
* name for the category.
|
* name for the category.
|
||||||
* model needed to initialise the custom view.
|
* model needed to initialise the custom view.
|
||||||
|
|
||||||
|
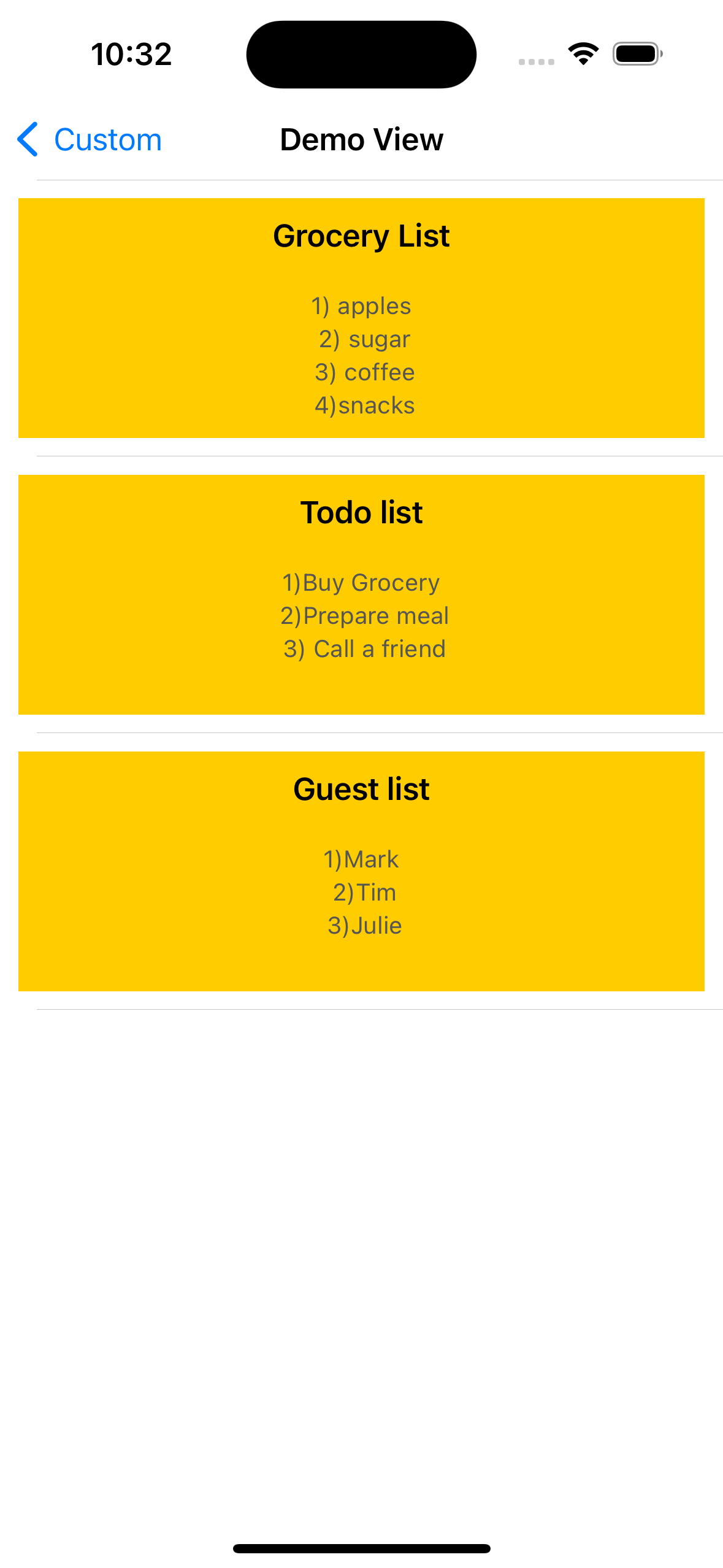
|
||||||
|
|
||||||
|
|
||||||
We can display view controllers in the catalog
|
We can display view controllers in the catalog
|
||||||
1. First create a custom destination that returns the view controller
|
1. First create a custom destination that returns the view controller
|
||||||
```
|
```
|
||||||
|
@ -203,7 +205,8 @@ struct CarouselCategory: Classification {
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
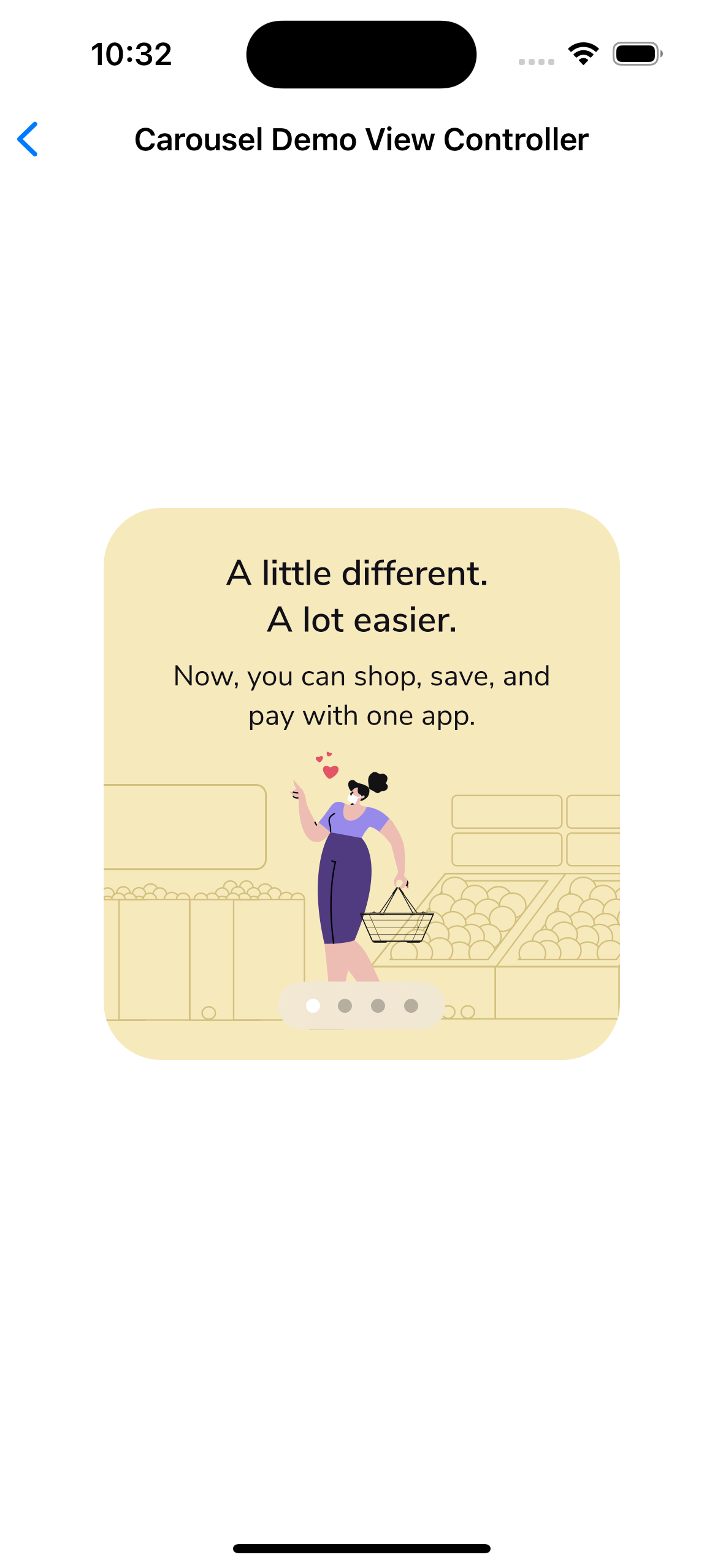
|
||||||
|
|
||||||
|
|
||||||
Contributing to Y—Catalog-Viewer
|
Contributing to Y—Catalog-Viewer
|
||||||
----------
|
----------
|
||||||
|
@ -270,6 +273,21 @@ When merging a pull request:
|
||||||
* Tag the corresponding commit with the new version (e.g. `1.0.5`)
|
* Tag the corresponding commit with the new version (e.g. `1.0.5`)
|
||||||
* Push the local tag to remote
|
* Push the local tag to remote
|
||||||
|
|
||||||
|
|
||||||
|
Requirements
|
||||||
|
----------
|
||||||
|
|
||||||
|
### Jazzy (documentation)
|
||||||
|
```
|
||||||
|
sudo gem install jazzy
|
||||||
|
```
|
||||||
|
|
||||||
|
Setup
|
||||||
|
----------
|
||||||
|
|
||||||
|
Open `Package.swift` in Xcode.
|
||||||
|
|
||||||
|
|
||||||
Generating Documentation (via Jazzy)
|
Generating Documentation (via Jazzy)
|
||||||
----------
|
----------
|
||||||
|
|
||||||
|
|
Loading…
Reference in New Issue